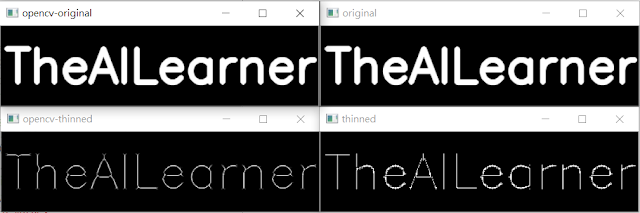
import numpy as np
import cv2
img = np.zeros((100,400),dtype='uint8')
font = cv2.FONT_HERSHEY_SIMPLEX
cv2.putText(img,'TheAILearner',(5,70), font, 2,(255),5,cv2.LINE_AA)
img1 = img.copy()
x=img.shape[0]
y=img.shape[1]
thin = np.zeros(img.shape,dtype='uint8')
def e(img2):
b=np.uint8(np.zeros((x,y)))
for i in range(1,x-1):
for j in range(1,y-1):
if (img2[i-1][j-1]==255 and img2[i-1][j+1]==255 and img2[i+1][j-1]==255 and img2[i+1][j+1]==255):
b[i][j]=255#侵蝕
return b
def o(img2):
c=np.uint8(np.zeros((x,y)))
for i in range(1,x-1):
for j in range(1,y-1):
if (img2[i-1][j-1]==255 and img2[i-1][j+1]==255 and img2[i+1][j-1]==255 and img2[i+1][j+1]==255):
c[i][j]=255#侵蝕
d=c.copy()
for i in range(1,x-1):
for j in range(1,y-1):
if (c[i][j]==255):
d[i][j]=255
d[i-1][j-1]=255
d[i+1][j-1]=255
d[i-1][j+1]=255
d[i+1][j+1]=255#膨脹
return d
while (cv2.countNonZero(img1)!=0): #細線化
erode = e(img1)
opening = o(erode)
subset = erode - opening
thin = cv2.bitwise_or(subset,thin)
img1 = erode.copy()
cv2.imshow('original',img)
cv2.imshow('thinned',thin)
# Create an image with text on it
img = np.zeros((100,400),dtype='uint8')
font = cv2.FONT_HERSHEY_SIMPLEX
cv2.putText(img,'TheAILearner',(5,70), font, 2,(255),5,cv2.LINE_AA)
img1 = img.copy()
# Structuring Element
kernel = cv2.getStructuringElement(cv2.MORPH_CROSS,(3,3))
# Create an empty output image to hold values
thin = np.zeros(img.shape,dtype='uint8')
# Loop until erosion leads to an empty set
while (cv2.countNonZero(img1)!=0):
# Erosion
erode = cv2.erode(img1,kernel)
# Opening on eroded image
opening = cv2.morphologyEx(erode,cv2.MORPH_OPEN,kernel)
# Subtract these two
subset = erode - opening
# Union of all previous sets
thin = cv2.bitwise_or(subset,thin)
# Set the eroded image for next iteration
img1 = erode.copy()
cv2.imshow('opencv-original',img)
cv2.imshow('opencv-thinned',thin)
cv2.waitKey(0)
cv2.waitKey(0)
cv2.destroyAllWindows()
留言
張貼留言